In our previous article, we introduced bash. In general, we made .sh samples. Now we will talk about other topics. Linux bash.
Table of Contents
Get value from user
The read keyword is used to get the value from the user.
#!/bin/sh
read
echo "Value:" $REPLY
To pass the received value to the variable, it will be sufficient to write the variable name to the read keyword.
#!/bin/sh
echo "What's your name?"
read NAME
echo "Welcome, $NAME"
It can be used in various parameters while getting values.
#!/bin/sh
read -p "Your Name:" NAME
read -sp "Your Password:" PASSWORD
echo
echo $NAME:$PASSWORD
Arithmetic Operators
The let keyword is used to perform arithmetic operations in Bash.
#!/bin/sh
read -p "First issue:" ISSUE1
read -p "Second issue:" ISSUE2
let RESULT=NUM1+NUM2
echo "Collection: "$RESULT
let RESULT=NUM1-NUM2
echo "Output: "$RESULT
let RESULT=NUMBER1*NUMBER2
echo "Multiplication: "$RESULT
let RESULT=NUM1/NUM2
echo "Split: "$RESULT
let RESULT=NUM1%NUM2
echo "Mode: "$RESULT
let RESULT=NUMBER1**NUMBER2
echo "Top: "$RESULT
let RESULT++
echo "Increment: "$RESULT
let RESULT--
echo "Reduce: "$RESULT
For arithmetic operations, expr and double brackets can also be used.
#!/bin/sh
A=50
B=40
echo `expr $A + $B`
echo $(($A + $B))
Conditional Expressions
The if structure is used to operate based on a condition or condition.
Its basic usage is as follows.
if [ <condition> ]
then
<commands>
fi
A simple example;
#!/bin/sh
A=2023
B=2023
if [ $A -eq $B ]
then
echo "$A ve $B equals."
fi
Conditions in conditional expressions are processed with the test command. For more examples use the man test command
Arithmetic Operators
-eq Equals.
-what is not equal.
-gt Big.
-ge equals Great.
-lt is small.
-le is equal to Small.
Text Operators
= is equal.
=! It is not equal.
-z Length is zero.
-n Length is not zero.
Logical Operators
! It is not.
-a and
-o Or
File and Directory Operators
-f Is the file special or ordinary?
-r Is the file readable?
-w Is the file writable?
-x Is the file executable?
-d File or directory?
-s Is the file empty or full?
-e Is there a file or not?
You can access detailed information with the man test command. If the condition is not met, it can be run in other commands with else.
#!/bin/sh
A=2023
B=1923
if [ $A -eq $B ]
then
echo "$A and $B are equal."
else
echo "$A and $B are not equal."
fi
If a condition yields more than two results, another condition can be specified with elif.
#!/bin/sh
A=2023
B=1923
if [ $A -gt $B ]
then
echo "$A is greater than $B."
elif [ $A -lt $B ]
then
echo "$A is less than $B."
else
echo "$A and $B are equal."
fi
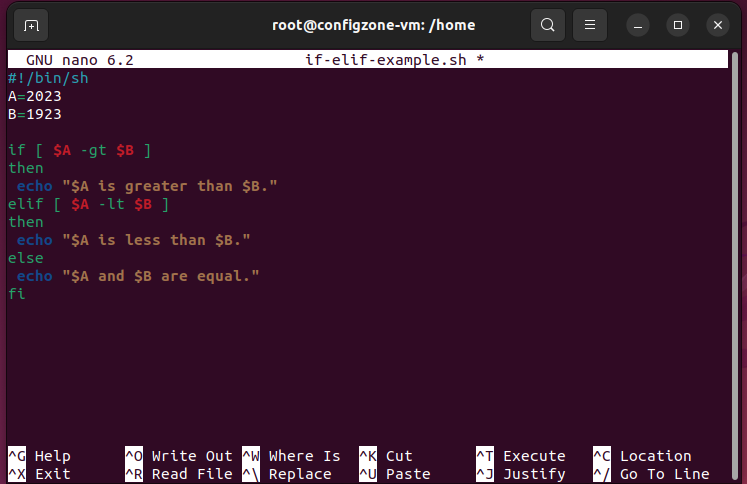
In almost all computer languages, logical operators are used to control and direct program flow. For example; If the username is Config and the password is Zone, if we want to write a script that says successfully logged in, we can use the -a or && logical operators.
#!/bin/sh
read -p "Your Name:" NAME
read -sp "Your Password:" PASSWORD
echo
if [ $NAME = "Config" ] && [ $PASSWORD= "ZONE" ]
#if [ $NAME = "Config" -a $PASSWORD= "ZONE" ]
then
echo "Successfully logged in."
else
echo "The username or password appears incorrect."
fi
Having more than one condition complicates the code. In these cases, the case structure can be used. In other programming languages it is known as switch-case. But basically, the working principle of this structure is the same in all programming languages.
#!/bin/sh
read -p "What day of the week:" DAY
case $DAY in
1) echo "Monday"
;;
2) echo "Tuesday"
;;
3) echo "Wednesday"
;;
4) echo "Thursday"
;;
5) echo "Friday"
;;
6) echo "Saturday"
;;
7) echo "Sunday"
;;
*) echo "Bad day"
;;
esac
Loops
For, while, and until loops are used for repetitive operations.
for variable in <list>
do
<commands>
done
The following command can be used to list array elements.
#!/bin/sh
CONTACTS=("Linus" "Stave" "Mark" "Cabbar");
for CONTACT in ${CONTACTS[*]}
do
echo $PERSON
done
The while usage is as follows.
#!/bin/sh
A=10
while [ $A -gt 0 ]
do
echo $A
A=$(($A - 1))
#A=`expr $A - 1`
done
The difference of the until loop from the while loop is that it runs as long as the condition is false.
#!/bin/sh
A=10
until [ ! $A -gt 0 ]
do
echo $A
A=$(($A - 1))
#A=`expr $A - 1`
done
Loops can also be nested.
#!/bin/sh
A=0
while [ "$A" -lt 10 ]
do
B="$A"
while [ "$B" -ge 0 ]
do
echo -n "$B"
((B--))
done
echo
((A++))
done
The continue keyword is used to skip the loop step.
#!/bin/sh
for NUMBER in {1..5}
do
if [ $NUM -eq 3 -o $NUM -eq 5 ]
then
continue;
fi
echo $NUM
done
The break keyword is used to terminate the loop.
#!/bin/sh
for NUMBER in {1..5}
do
if [ $NUM -eq 3 ]
then
break;
fi
echo $NUM
done
Functions
Function structure is used to organize commands and reuse written commands. Functions also make the script we write more understandable. In addition, we can make the codes that we do the same operations function and use them in many places. Thus, we do more with less lines of code.
function_name() {
<commands>
}
or
function function_name {
<commands>
}
Function names are understandable and it is helpful to use a name that identifies the function.
#!/bin/sh
name_print() {
echo "Config Zone"
}
name_print
name_print
To return a value, the return keyword is used and $? , the returned value is reached.
#!/bin/sh
gather () {
return `expr $1 + $2`
}
gather 10 20
echo $?
In my previous article, we introduced bash programming. You can access that article here.
One thought on “Linux Bash-2”